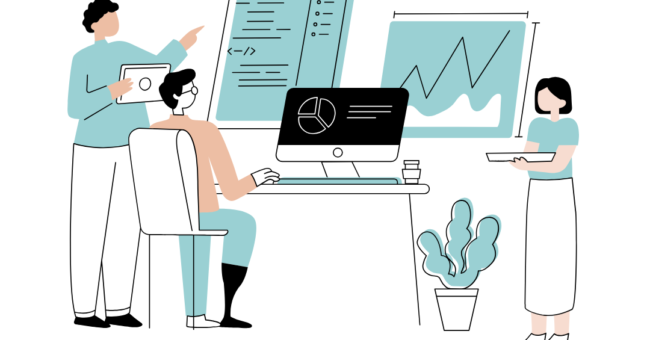
Palindrome Number
Example 1:
Input: x = 121
Output: true
Explanation: 121 reads as 121 from left to right and from right to left.
Example 2:
Input: x = -121
Output: false
Explanation: From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
Example 3:
Input: x = 10
Output: false
Explanation: Reads 01 from right to left. Therefore it is not a palindrome.
public class Solution {
public boolean isPalindrome(int x) {
// Convert integer to string
String s = Integer.toString(x);
// Use two pointers to check if the string is a palindrome
int left = 0, right = s.length() - 1;
while (left < right) {
// If characters at current positions don't match, it's not a palindrome
if (s.charAt(left) != s.charAt(right)) {
return false;
}
// Move the pointers towards the center
left++;
right--;
}
// If the loop completes without returning false, the number is a palindrome
return true;
}
public static void main(String[] args) {
Solution solution = new Solution();
int x = -121;
boolean result = solution.isPalindrome(x);
System.out.println("Output: " + result);
}
}
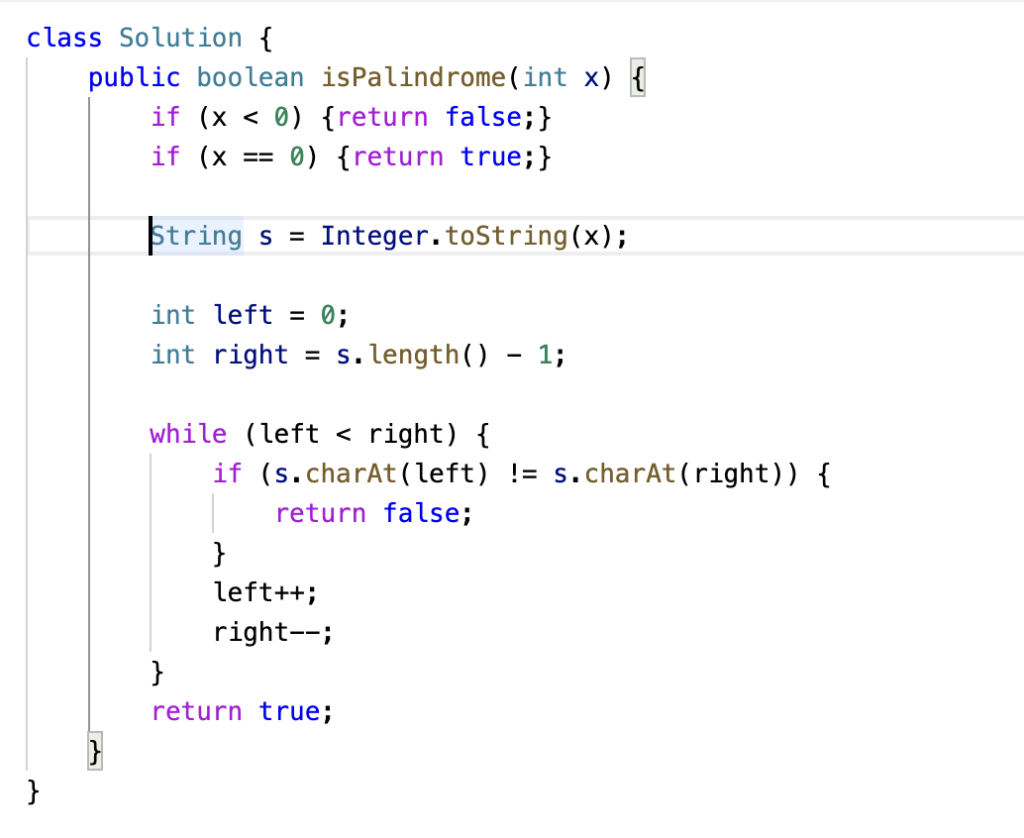
Explanation:-
- isPalindrome Method:
- This method takes an integer
x
as input and returns a boolean value indicating whetherx
is a palindrome or not. - It first converts the integer
x
to a strings
using theInteger.toString(x)
method. This allows us to easily work with individual digits of the number. - It initializes two pointers,
left
andright
, to traverse the strings
.left
starts from the beginning (index 0) of the string, andright
starts from the end (indexs.length() - 1
) of the string.
- Looping through the String:
- The method enters a while loop that continues as long as
left
is less thanright
. This loop compares characters from both ends of the string towards the center. - At each iteration, it checks if the character at position
left
in the string is equal to the character at positionright
. If they are not equal, it means the number is not a palindrome, so it returnsfalse
. - If the characters are equal, the pointers are moved towards the center by incrementing
left
and decrementingright
.
- Returning the Result:
- If the loop completes without returning
false
, it means all characters from both ends matched, indicating that the number is a palindrome. In this case, it returnstrue
.
- Main Method:
- The
main
method is where the program execution starts. - An instance of the
Solution
class is created. - An integer
x
with a value of-121
is declared. - The
isPalindrome
method is called withx
as an argument, and the result is stored in theresult
variable. - Finally, the result is printed to the console.
This code effectively checks whether the given integer is a palindrome by converting it to a string and comparing characters from both ends towards the center.