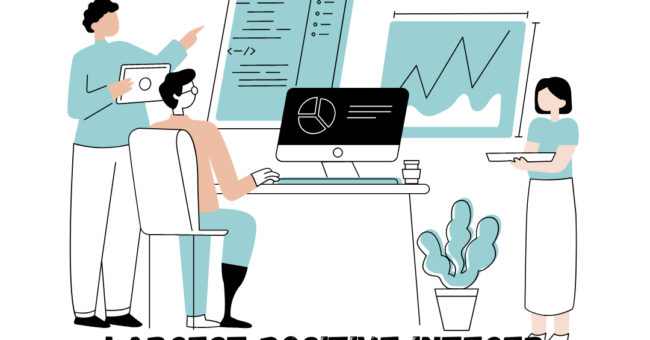
class Solution {
public int findMaxK(int[] nums) {
HashSet<Integer> numSet = new HashSet<>();
int maxK = -1;
// Adding all numbers to the set
for (int num : nums) {
numSet.add(num);
}
// Iterating through the array to find the largest positive integer k
for (int num : nums) {
if (numSet.contains(-num) && num > 0) {
maxK = Math.max(maxK, num);
}
}
// Returning the result
return maxK;
}
}
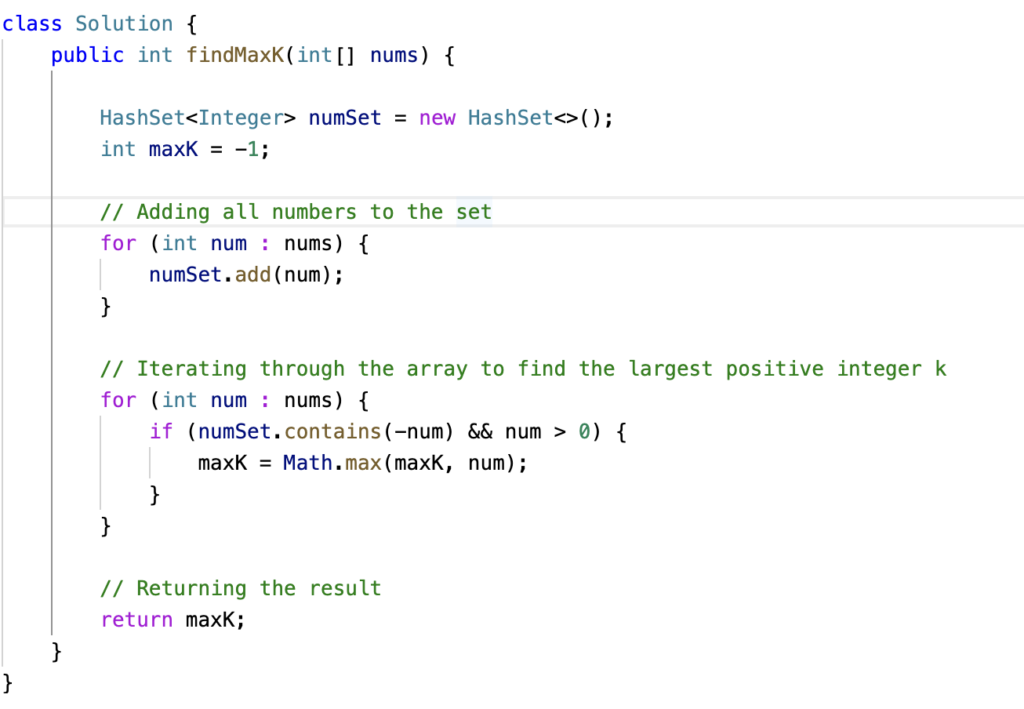
This Java code defines a method findMaxK
within a class Solution
. The purpose of this method is to find the largest positive integer k
such that its negative counterpart -k
exists in the given array nums
.
Here’s a breakdown of how the code works:
- Initialization:
HashSet<Integer> numSet = new HashSet<>();
creates a HashSet to store unique integers from the input array.int maxK = -1;
initializes a variablemaxK
to-1
. This variable will hold the largest positive integerk
found in the array.
- Adding numbers to the set:
- The first loop iterates through each element
num
in thenums
array. - Inside the loop, each
num
is added to thenumSet
. This ensures that the HashSet contains unique elements from the input array.
- Finding the largest positive integer
k
:
- The second loop iterates through each element
num
in thenums
array again. - For each
num
, it checks if its negative counterpart-num
exists in thenumSet
(i.e., if-num
is present in the array). - Additionally, it checks if
num
is positive (i.e.,num > 0
). - If both conditions are true (i.e., if
-num
exists andnum
is positive), it updatesmaxK
to the maximum of its current value andnum
usingMath.max(maxK, num)
. This ensures thatmaxK
holds the largest positive integerk
encountered so far in the array.
- Returning the result:
- After looping through all elements in the array, the method returns the value of
maxK
, which represents the largest positive integerk
found in the array for which its negative counterpart exists.
Overall, this method efficiently finds the largest positive integer k
such that -k
exists in the given array. If no such k
is found, the method returns -1
.